4.1. Delegation¶
4.1.1. Purpose¶
Demonstrate the Delegator pattern, where an object, instead of performing one of its stated tasks, delegates that task to an associated helper object. In this case TeamLead professes to writeCode and Usage uses this, while TeamLead delegates writeCode to JuniorDeveloper’s writeBadCode function. This inverts the responsibility so that Usage is unknowingly executing writeBadCode.
4.1.2. Examples¶
Please review JuniorDeveloper.php, TeamLead.php, and then Usage.php to see it all tied together.
4.1.3. UML Diagram¶
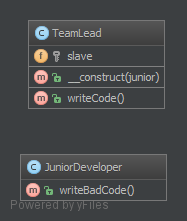
4.1.4. Code¶
You can also find these code on GitHub
Usage.php
1 2 3 4 5 6 7 8 9 | <?php
namespace DesignPatterns\More\Delegation;
// instantiate TeamLead and appoint to assistants JuniorDeveloper
$teamLead = new TeamLead(new JuniorDeveloper());
// team lead delegate write code to junior developer
echo $teamLead->writeCode();
|
TeamLead.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | <?php
namespace DesignPatterns\More\Delegation;
/**
* Class TeamLead
* @package DesignPatterns\Delegation
* The `TeamLead` class, he delegate work to `JuniorDeveloper`
*/
class TeamLead
{
/** @var JuniorDeveloper */
protected $slave;
/**
* Give junior developer into teamlead submission
* @param JuniorDeveloper $junior
*/
public function __construct(JuniorDeveloper $junior)
{
$this->slave = $junior;
}
/**
* TeamLead drink coffee, junior work
* @return mixed
*/
public function writeCode()
{
return $this->slave->writeBadCode();
}
}
|
JuniorDeveloper.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <?php
namespace DesignPatterns\More\Delegation;
/**
* Class JuniorDeveloper
* @package DesignPatterns\Delegation
*/
class JuniorDeveloper
{
public function writeBadCode()
{
return "Some junior developer generated code...";
}
}
|
4.1.5. Test¶
Tests/DelegationTest.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | <?php
namespace DesignPatterns\More\Delegation\Tests;
use DesignPatterns\More\Delegation;
/**
* DelegationTest tests the delegation pattern
*/
class DelegationTest extends \PHPUnit_Framework_TestCase
{
public function testHowTeamLeadWriteCode()
{
$junior = new Delegation\JuniorDeveloper();
$teamLead = new Delegation\TeamLead($junior);
$this->assertEquals($junior->writeBadCode(), $teamLead->writeCode());
}
}
|