2.10. Registry¶
2.10.1. Purpose¶
To implement a central storage for objects often used throughout the application, is typically implemented using an abstract class with only static methods (or using the Singleton pattern)
2.10.2. Examples¶
- Zend Framework 1:
Zend_Registry
holds the application’s logger object, front controller etc. - Yii Framework:
CWebApplication
holds all the application components, such asCWebUser
,CUrlManager
, etc.
2.10.3. UML Diagram¶
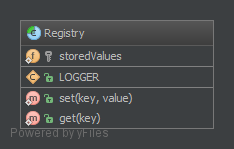
2.10.4. Code¶
You can also find these code on GitHub
Registry.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 | <?php
namespace DesignPatterns\Structural\Registry;
/**
* class Registry
*/
abstract class Registry
{
const LOGGER = 'logger';
/**
* @var array
*/
protected static $storedValues = array();
/**
* sets a value
*
* @param string $key
* @param mixed $value
*
* @static
* @return void
*/
public static function set($key, $value)
{
self::$storedValues[$key] = $value;
}
/**
* gets a value from the registry
*
* @param string $key
*
* @static
* @return mixed
*/
public static function get($key)
{
return self::$storedValues[$key];
}
// typically there would be methods to check if a key has already been registered and so on ...
}
|
2.10.5. Test¶
Tests/RegistryTest.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <?php
namespace DesignPatterns\Structural\Registry\Tests;
use DesignPatterns\Structural\Registry\Registry;
class RegistryTest extends \PHPUnit_Framework_TestCase
{
public function testSetAndGetLogger()
{
Registry::set(Registry::LOGGER, new \StdClass());
$logger = Registry::get(Registry::LOGGER);
$this->assertInstanceOf('StdClass', $logger);
}
}
|